Test Automation using Mocha, Chai, and TypeScript
Today we will be setting up a test framework using one of the famous JavaScript tool named Mocha and Chai Assertion library with TypeScript as the programming language.

Today we will be setting up a test framework using one of the famous JavaScript tool named Mocha and Chai Assertion library with TypeScript as the programming language.
WHAT IS MOCHA?
Mocha is a feature-rich JavaScript test framework running on Node.js and in the browser, making asynchronous testing simple and fun. Mocha tests run serially, allowing for flexible and accurate reporting, while mapping uncaught exceptions to the correct test cases.
Reference: https://mochajs.org
WHAT IS CHAI?
Chai is a BDD / TDD assertion library for node and the browser that can be delightfully paired with any javascript testing framework.
Reference: https://www.chaijs.com/
Let go through step by step process of creating automation framework using Mocha, Chai, and TypeScript. At the end of this tutorial, you will be having knowledge of setting up the framework from scratch. Follow below steps one by one, and if you know any step you can skip it and move to next:
Open Visual Studio Code
Create a folder named mocha-chai-typescript
Create a file named .gitignore and add below content
Create a file named tslint.json and add below content
Create a file named package.json and add below content
Now go to your Project root directory which is mocha-chai-typescript and install dependency by running the command.
npm install chai mocha ts-node typescript --save
Ignore any warning you see.

Now install dev dependency run command
npm install @angular/compiler @angular/core @types/chai @types/mocha codelyzer rimraf rxjs tslint typings zone.js --save-dev
Ignore any warning you see.
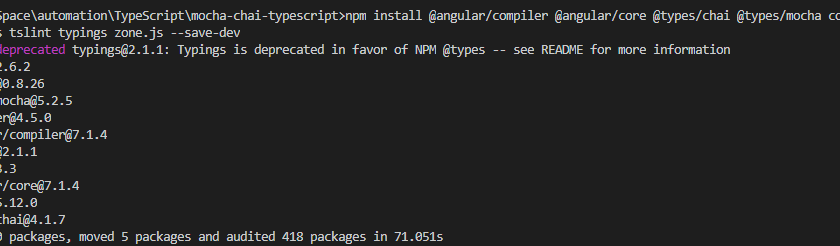
Create a folder named page-objects
Create a file named first-test.po.ts and add below content
export function hello() { return 'Hello world!'; } export default hello;
Now create a folder named test-suite
Create a file named first-test.spec.ts and add below content
import { hello } from '../page-objects/first-test.po'; import { expect } from 'chai'; import 'mocha'; describe('First Hello World Test: ', () => { it('Should return Hello World', () => { const result = hello(); expect(result).to.equal('Hello world!'); }); })
Now lets run the test
npm test
You can see the results in console.
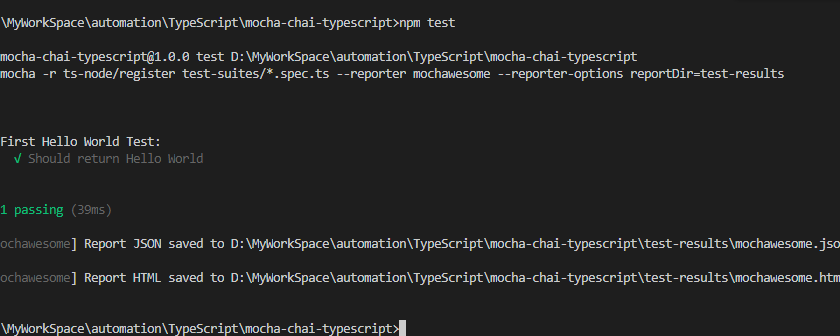
Also, you will get html report generated in test-results folder using mochawesome

CODE REPOSITORY
The sample framework is hosted on GitHub: mocha-chai-typescript
Have a suggestion or found a bug? Fork this project to help make this even better.
Star the repo and follow me to get latest updates
WHAT DO YOU THINK?
Did this work for you?
Could I have done something better?
Have I missed something?
Please share your thoughts using comments on this post. Also, let me know if there are particular things that you would enjoy reading further.
Cheers!